Creating Fair But Random Combat
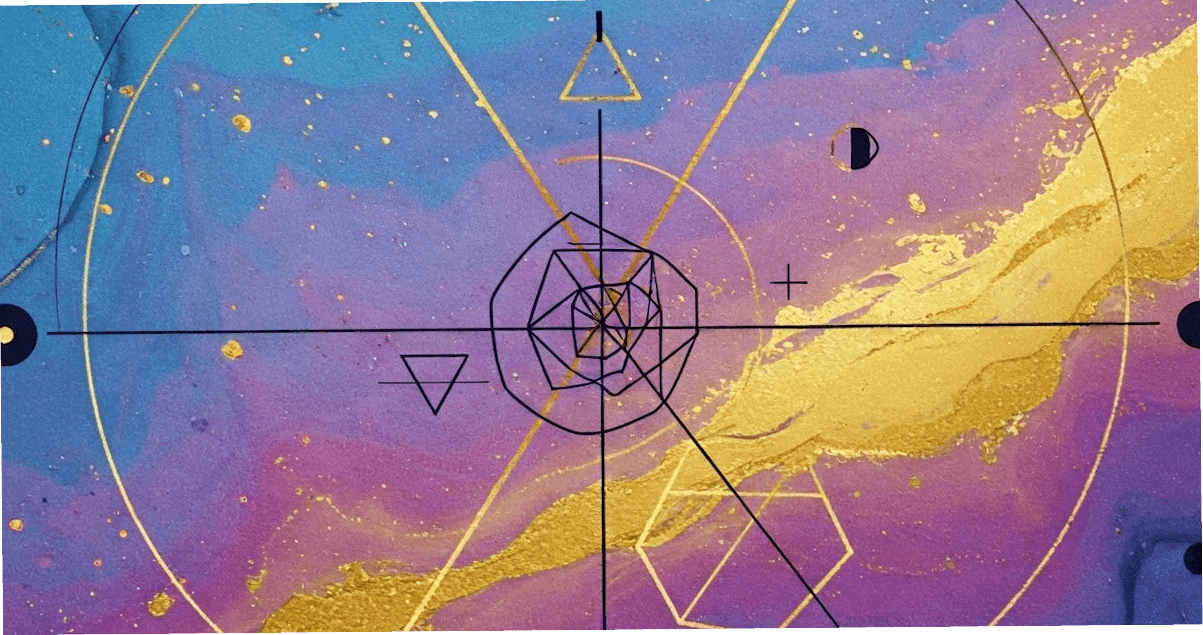
Creating Fair but Random Combat
Combat Revamp: Making Battles More Engaging
When I started building Delvers Inc, I originally thought that combat would take a back seat. Early iterations of the combat system used a more 'idle' game mechanic where combat was both auto-resolved as well as auto-hit, I had a weird number crunching scheme to try and adjust for defensive modifiers but it never felt quite right. Like something stuck in my teeth that I couldn't get out but kept ignoring. Battles felt too same-y all the time and the outcome was never in doubt.
The problem wasn't that the system was broken; it was doing exactly what I wanted at the start. The problem was that it didn't feel right to play, didn't feel engaging. One night I happened upon a video discussing the two types of "random" in games (this one if you're interested in watching it yourself: The Two Types of Random in Game Design). This got me considering that the auto-battle/auto-hit mechanic wasn't right and that I had gone down the wrong path. This feeling drove me to completely revamp the combat system, searching for that perfect balance between randomness (which generates exciting moments) and consistency (which creates perceived fairness and help avoid rage quitting).
This journey led me to develop a "Bounded Combat Resolver"; a system that maintains the excitement and danger of random outcomes while ensuring players aren't subject to the extreme and often frustrating outliers that pure randomness creates AND removes the same-y-ness (is that a word?) of the auto-hit system. Inspired by and modelled after HeroScapes Skull & Shield dice system (Rules PDF here if you aren't familiar with it: HeroScape Basic Game Guide), it has the side benefit of making the math easier and balancing of combat more straightforward.
Introduction: When Random Isn't Fun
Picture this scenario: Your character has a 95% chance to hit. You're one attack away from defeating the boss. You swing... and miss. You try again... and miss. A third attempt... and miss again.
Mathematically, this streak of bad luck is entirely possible, the chance of missing three times in a row with a 95% hit rate is low but never 0, rare but not impossible. However, when it happens to a player, it doesn't feel random; it feels targeted at the player or worse yet...broken.
- Pure randomness creates memorable moments when the odds turn in our favor
- Pure randomness creates rage-quit moments when the odds turn against us
Unfortunately, Players remember negative outcomes much more vividly than positive ones. It's just human nature.
The goal of a bounded combat resolver isn't to eliminate randomness but to tame its extreme edges. It's about creating a system where the highs and lows still exist, but extreme outliers (especially negative ones) are far less common.
The Problem with Traditional RNG in Combat Systems
The Reality vs. Perception Gap
Traditional random number generation (RNG) systems use flat probability distributions, each outcome within the range has an equal chance of occurring. This creates a fundamental disconnect between mathematical reality and player perception.
Traditional RNG often feels unfair because:
- Humans are pattern-seeking creatures — We instinctively look for patterns even in randomness
- Negativity bias dominates perception — We notice and remember negative outcomes more strongly than positive ones
- Flat distributions can enable extreme outcomes — With pure randomness, nothing prevents multiple unlikely events from occurring in sequence. They are unlikely not impossible.
In a purely random system, streaks are not just possible, they're inevitable. A 90% hit chance means you'll miss 1 in 10 times on average, but nothing prevents those misses from clustering together. When this happens, players don't blame mathematics; they blame the game. 'The game is cheating', 'The RNG gods hate me', 'This stupid game is broken! <throws tablet at the wall>'
The human brain isn't wired to intuitively understand probability. When we see a 90% hit chance, we expect something closer to "hit 9 times, then miss once, repeat", not the clumpy, streaky reality of true randomness.
The Rule of Cool: Prioritizing Player Experience
When designing combat systems, there's a critical hierarchy of priorities to consider:
- Is it fun? — Above all else, does the system create enjoyable gameplay moments?
- Does it feel fair? — Do players feel like outcomes reflect their decisions and character builds?
- Is it mathematically sound? — Does the system make sense within your game's ecosystem?
Note the order here, math purity and true randomness take a backseat to player enjoyment. This leads us to what a good Dungeon Master would call "the rule of cool": bend some rules under the pretext of player enjoyment.
A few principles emerge from this priority list:
- Perceived fairness trumps actual mathematical fairness
- Consistency builds trust in your game's systems
- Reliable outcomes create value perception — players feel their time (and potentially monetary!) investment matters
A bounded combat system addresses this by ensuring that, while randomness still exists, it operates within constraints/bounds that align with what the player expects.
Bounded Randomness: The Best of Both Worlds?
Alright already, stop beating the drum....so what IS Bounded Randomness?
Bounded randomness is an approach that constrains random outcomes to fall within a certain range around an expected value. Think of it as putting guardrails on your RNG system.
- They use bell curves instead of flat distributions
- They establish minimum and maximum thresholds based on statistical expectations
- They still produce varied results but prevent extreme outliers that cause rage quitting
To put it a different way, imagine plotting 1,000 combat results on a graph:
- With traditional RNG: Results would spread evenly across the entire spectrum, with some clusters of extremely good or bad outcomes
- Bounded RNG: Results would cluster around the expected value, with fewer examples at the extreme ends
Building The Bounded Combat Resolver
Let's look at how to implement a bounded combat resolver using the approach developed for Delvers Inc.
The Core Algorithm
The algorithm uses a few key steps:
- Calculate expected outcomes based on character stats
- Determine acceptable deviation ranges that scale with the input values
- Generate a normally distributed random value using the Box-Muller transform
- Ensure minimum satisfying outcomes even on "failures"
Here's a simplified version of the core damage calculation function but first some background on the combat mechanic itself:
- Every mob in the game has an attackPower and every mob in the game has a defensePower.
- Each point of power is essentially 1 "d6 die roll" where 4+ is a 'success'
- There is no real "to hit" per se, but if a monsters defense success is higher then your attack success then ... you missed.
public int CalculateDamage(int attackPower, int defensePower, float criticalChance = 0.1f)
{
// Calculate base expected damage
var baseDamage = Math.Max(attackPower - defensePower, 0);
// Calculate bounded attack and defense values
var attackSuccesses = CalculateBoundedSuccesses(attackPower);
var defenseSuccesses = CalculateBoundedSuccesses(defensePower);
// Actual damage is attack successes minus defense successes
// Remember, this system was inspired by the board game HeroScape
var boundDamage = Math.Max(attackSuccesses - defenseSuccesses, 0);
// Apply boundaries to keep results within expectations
// This is a boundary ontop of the boundary because while we used
// bounded success rolls, even that could lead to problems with extreme outliers
// so we bound the damage in guardrails as well
var minAcceptableDamage = Math.Max(Math.FloorToInt(baseDamage * 0.7f), attackPower > 0 ? 1 : 0);
var maxAcceptableDamage = Math.CeilToInt(baseDamage * 1.3f);
boundDamage = Math.Clamp(boundDamage, minAcceptableDamage, maxAcceptableDamage);
return boundDamage;
}
The "magic" happens in the CalculateBoundedSuccesses
method:
private int CalculateBoundedSuccesses(int diceCount)
{
// Calculate expected number of successes
// Expected success rate in our case is 50% (4+ on a D6)
var expectedSuccesses = diceCount * ExpectedSuccessRate;
// Calculate allowed deviation based on dice count
var deviation = Math.Sqrt(diceCount) * 0.5f;
var minSuccesses = Math.Max(0, Math.FloorToInt(expectedSuccesses - deviation));
var maxSuccesses = Math.Min(diceCount, Math.CeilToInt(expectedSuccesses + deviation));
// Generate a normal distribution value centered around the expected value
var normalRandom = CountBoundedSuccesses(expectedSuccesses, deviation / 2);
// Round and clamp within boundaries
var successes = Math.RoundToInt(normalRandom);
successes = Math.Clamp(successes, minSuccesses, maxSuccesses);
return successes;
}
So, here the system:
- Calculates expected successes based on the dice count
- Establishes boundaries based on the square root of the dice count
- Uses a normal distribution to generate results clustered around the expected value
- Clamps final results to ensure they stay within the established boundaries
The point here is that, as the power level (dice count) increases, the allowed deviation increases as well, but at a much slower rate. This creates a system where high-level characters have more consistent outputs than low-level ones.
Fair AND Fun
The bounded combat resolver sounds a bit sketchy I know. It raised some eyebrows on me when I first encountered it as well, BUT it's a player experience solution. By placing constraints on randomness without eliminating it entirely, we create combat that feels both fair and exciting. We maintain the players understanding that the time they are putting in to make their characters better is worth it and that the game isn't cheating them out of their fair shake.
- Players trust that their character builds matter
- Each combat encounter maintains excitement without frustration
- The system scales naturally with character progression
In the end, this is about finding that sweet spot between chaos and control. A bounded system acknowledges that while unpredictability creates excitement, extreme outliers create frustration. The goal isn't to eliminate randomness, it's to keep it in check so that it's just enough that players always feel like they're getting a fair shake and that the game isn't cheating, even when the dice don't roll in their favor.